How JWT Authentication Works
Learn how JWT authentication works and how you can use it to secure your APIs.
Hakan Shehu
April 17, 2024
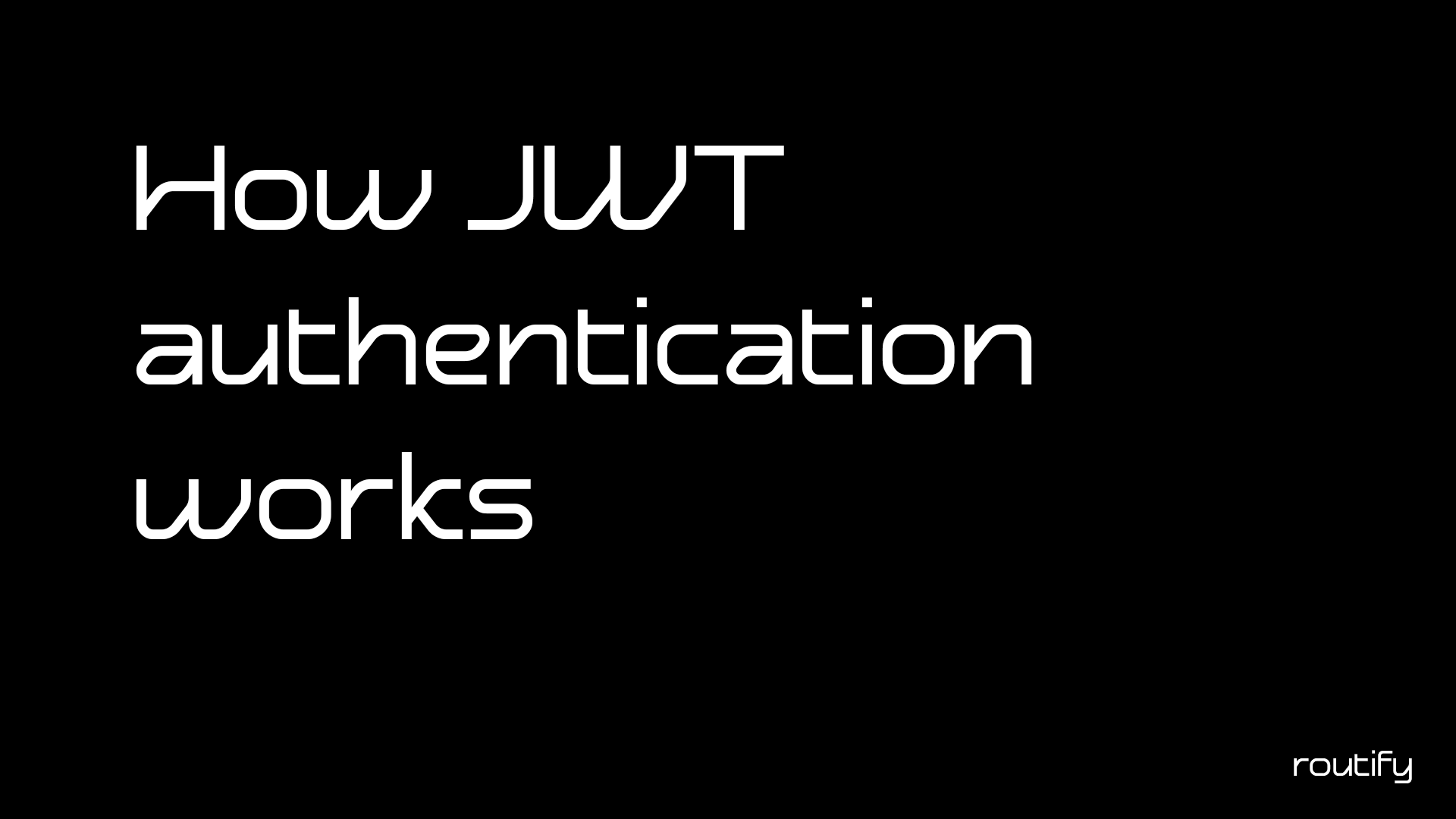
A JSON Web Token (JWT) is a compact, URL-safe token that represents claims between two parties. It is composed of three parts:
- Header: Contains the type of token (JWT) and the signing algorithm being used (e.g., HMAC SHA256).
- Payload: Contains the claims. This is the part of the token where you can store user data and any other relevant information. Common claims include user ID, username, and roles.
- Signature: Used to verify the token's authenticity. It is created by encoding the header and payload, and then signing them using a secret key or a public/private key pair.
Structure of a JWT
A typical JWT looks like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VybmFtZSI6ImpvaG5kb2UiLCJpYXQiOjE1MTYyMzkwMjJ9.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
This string consists of the header, payload, and signature separated by dots.
How JWT Authentication Works
JWT authentication typically follows these steps:
- Client Login: The client sends a login request with their credentials (e.g., username and password) to the server.
POST /login
{
"username": "johndoe",
"password": "password123"
}
-
Server Validation: The server verifies the credentials. If valid, it creates a JWT containing the user's information and other claims.
-
Token Generation: The server signs the JWT using a secret key and sends it back to the client.
{
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9..."
}
-
Client Storage: The client stores the JWT, typically in local storage or cookies, for use in subsequent requests.
-
Authenticated Requests: For future requests, the client includes the JWT in the HTTP headers.
GET /protected
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
- Server Verification: The server verifies the JWT’s signature and extracts the claims. If valid, it processes the request; if not, it responds with an error.
Benefits of JWT Authentication
- Stateless: JWTs are self-contained, meaning the server does not need to store session information, making it easier to scale applications.
- Security: JWTs can be signed and encrypted to ensure the integrity and confidentiality of the data they contain.
- Compact: The compact nature of JWTs makes them easy to pass in URL parameters, headers, or within the body of a POST request.
- Cross-Domain: JWTs are ideal for applications with multiple domains as they can be easily shared between them.